Asynchronous loading of external CSS and JS resources helps to increase the speed of website loading and display of web pages in the browser, ensuring that files are loaded and executed in the background without blocking rendering. The time it takes to Load CSS Asynchronously is short.
What is Asynchronous Loading?
Delays accompany the display page in the browser ( Render Blocking ) whenever the browser detects external CSS and JS resources in tags link and script. This leads to the fact that for some time, the user is waiting for the display of the web page on the screen until the files that prevent it from being displayed are loaded and executed.
For example, a block of a headweb page has the following content:
<head> <title> Bootstrap Agency Template </title> <link rel = "stylesheet" href = "/css/bootstrap.css"> <script src = "/js/jquery-1.11.3.min.js" > </script> <script src = "/js/bootstrap.js" > </script> </head>
The results of the analysis of this page in the PageSpeed Insights service will necessarily contain the recommendation “Remove the code javascript and CSSblocking the display of the top of the page “:
This means that the page will not start rendering in the browser until all external CSS and JS files specified in the tag head have been loaded and applied.
To avoid page rendering delays, CSS and JavaScript files are loaded asynchronously to ensure that the page is rendered non-stop in the browser.
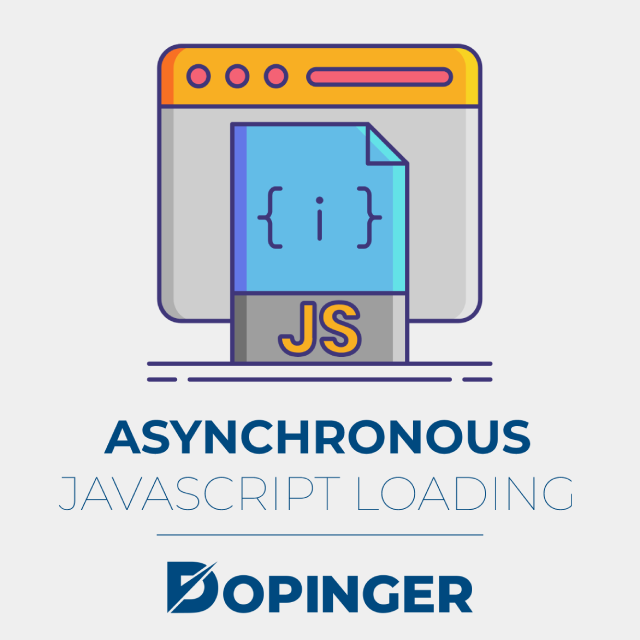
Asynchronous JavaScript Loading
JS files can be loaded asynchronously using attributes defer or an async tag script. In both cases, loading JS files does not delay the display of the page. The difference lies at the moment the script is executed.
SCRIPT Tag Without Attributes (Synchronous JS loading)
<head> <script src = "/jquery.js" > </script> </head>
If the tag script is applied without attributes deferor async, then loading the script delays the process of displaying (rendering) the page until it is loaded and executed.
SCRIPT Tag With ASYNC Attribute (Asynchronous JS Loading)
<head> <script src = "/jquery.js" async > </script> </head>
The attribute asyncprovides an asynchronous loading of an external JS file: the file will be loaded and executed in the background without delaying the page display.
When using tags scriptwith an attribute async, keep in mind that the order of the execution of scripts (if there are several JS files) is not preserved: they will be executed when they have finished loading, regardless of their order the HTML code. This can lead to errors and scripts failing.
<head> <! -bootstrap.js using jquery.js will not function as scripts are executed asynchronously -> <script src = "/jquery.js" async > </script> <script src = "/bootstrap.js" async > </script> </head>
The solution is to combine the code of all JS files into one or use the defer attribute if the problem concerns only external files.
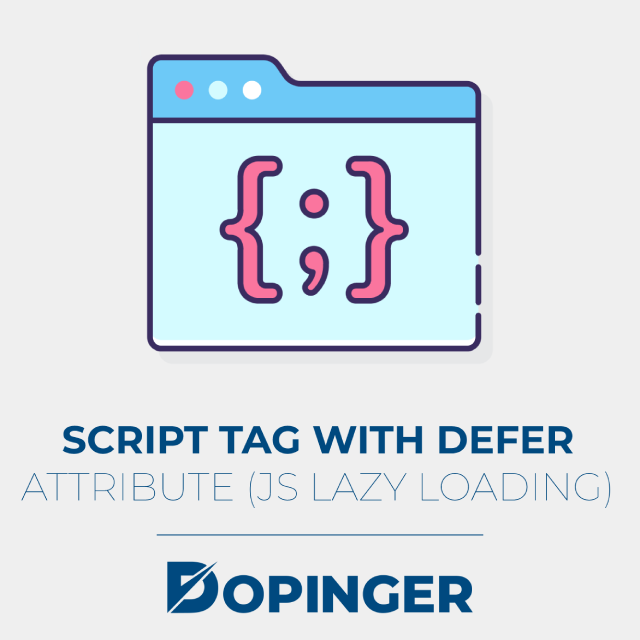
SCRIPT Tag With DEFER Attribute (JS Lazy Loading)
<head> <script src = "/jquery.js" defer > </script> </head>
The attribute deferprovides lazy loading of an external JS file: the file will be loaded without delaying the page display and executed when rendering is complete.
Also, the use of the attribute defer, unlike async, preserves the sequence of execution of external scripts:
<head>
<script src = "/jquery.js" defer > </script> <script src = "/bootstrap.js" defer > </script> </head>
It should be borne in mind that the attribute defer (as well as async) does not postpone the execution of scripts embedded in the page: they will be analyzed by the browser, and if the JS library file they use is not loaded and executed, then the scripts will be ignored:
<head> <script href = "/jquery.js" defer > </script> <script> // this script uses jquery.js and the browser will ignore it // because jquery.js will be deferred
$ ( document ). ready ( function () {
$ ( 'head' ). append ( '<link rel = "stylesheet" href = "/ style.css">' ); }) </script> </head>
For Sites on CMS
An excellent solution providing asynchronous loading scripts for sites on the Joomla, the WordPress, Drupal is a plug- JCH the Optimize, allowing not only add attributes asyncand defertags script, but also to integrate all external JS-files into one, and place them before the closing body.
Asynchronous CSS Loading
Loading and processing external style files by the browser are also accompanied by blocking page rendering, which can be avoided by loading them asynchronously. The tricky part is that you cannot implement asynchronous CSS loading using tag attributes, unlike JS files. You can solve the problem in different ways using JavaScript.
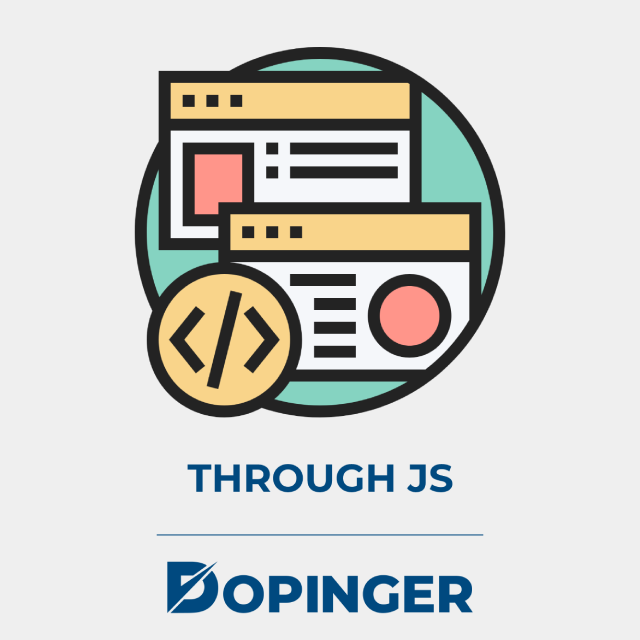
Through JS
You can use JavaScript to load external CSS files asynchronously without using third-party libraries.
In order for you to do this, you can use the following JS function:
function asyncCSS ( href ) { var css = document . createElement ( 'link' );
css . rel = "stylesheet" ;
css . href = href ;
document . head . appendChild ( css ); }
After declaring a function in the code, you need to call it the number of times corresponding to the number of CSS files:
// The URL of the file is specified in brackets
asyncCSS ( 'style1.css' );
asyncCSS ( 'style2.css' );
asyncCSS ( 'style3.css' );
To use this method, place the function and its call (s) in a tag scriptbefore the closing tag body:
<body>
<script> function asyncCSS ( href ) { var css = document . createElement ( 'link' );
css . rel = "stylesheet" ;
css . href = href ;
document . head . appendChild ( css ); }
asyncCSS ( 'style1.css' );
asyncCSS ( 'style2.css' );
asyncCSS ( 'style3.css'
); </script> </body>
With jQuery
If your page uses the jQuery JS library , you can use the following code to Load CSS Asynchronously, with its files:
<script>
$ ( document ). ready ( function () {
$ ( 'head' ). append ( '<link rel = "stylesheet" href = "/ style.css" />' ); }) </script>
This script determines that when the page completes the render, the head-specified tag link defining the CSS file must be in the block, which will ensure its asynchronous loading.
If you need to load multiple style files asynchronously, you need to list them in the JS function:
<script>
$ ( document ). ready ( function () {
$ ( "head" ). append ( "<link rel = 'stylesheet' href = 'style1.css'>" );
$ ( "head" ). append ( "<link rel =' stylesheet 'href =' style2.css'> " );
$ ( " head " ). append ( " <link rel = 'stylesheet' href = 'style3.css'> " ); }) </script>
It is important to understand that jQuery must preload to execute the above script. The tag scriptcontaining the link to the jQuery file must precede the specified script. Otherwise, it will not work, and the styles will not start setting to the page:
<script href = "/jquery.js" > </script> <script>
$ ( document ). ready ( function () {
$ ( 'head' ). append ( '<link rel = "stylesheet" href = "/ style.css">' ); }) </script>
At the same time, if the JS file with jQuery is loading asynchronously (using the attribute asyncor defer), then the script for asynchronous CSS loading will also not work since the browser parser already bypasses this script, having no data to execute it ( jQuery at this moment will be only at the loading stage):
<
script href = "/jquery.js"
async > < /script> <script> / / This script will not be executed because when jQuery loads asynchronously, the browser will
continue processing the page data
$(document).ready(function() {
$('head').append('<link rel = "stylesheet" href = "/ style.css">');
}) < /script>
To load JavaScript(jQuery) and CSS asynchronously, you can use the following trick:
<
script >
var js = document.createElement('script');
js.src = 'jquery.js'; // quoted link to jQuery file
document.head.appendChild(js);
js.onload = function() {
$('head').append('<link rel = "stylesheet" href = "/ style.css">');
}; < /script>
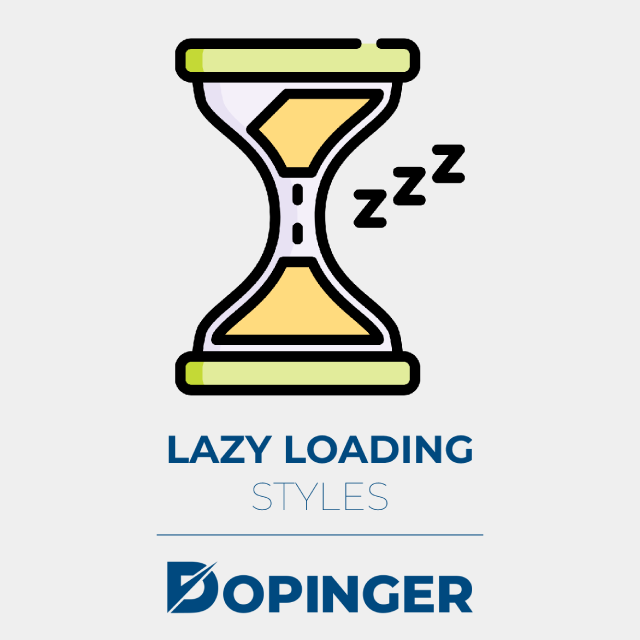
Lazy Loading Styles
Each of the methods can postpone the loading of styles; as a result, the page first renders without applying them, after which the styles are abruptly setting, transforming the page.
To avoid this unwanted visual effect, you need to extract a number of CSS properties from the style files for the HTML elements that form the page’s visible top and place them in a tag style within a blockhead.
A quick heads-up as a summary
Functions of JS and CSS
JS and CSS files accelerate the loading and display of site pages.
ASYNC + Defer
JS files are provided using attributes async(eager code execution) or defer(deferred execution).
Asyncand + Defermay
JS library files through the attribute asyncand defermay result in non-execution of embedded scripts using these libraries.
Non-execution of external JS
JS library files through the attribute asynccan also result in non-execution of external JS files using methods of these libraries.
Loading CSS Asynchronously
To Load CSS Asynchronously, their files start implementing while using.
How to Load CSS Asynchronously in Short
In this article, we talked about how to load CSS Asynchronously.
Frequently Asked Questions About
You can avoid page rendering delays when CSS and JavaScript files are loading asynchronously. This action ensures that the page renders non-stop in the browser.
When loading JS files, they do not delay the display of the page.
Yes, there is a solution; it starts by combining the code of all JS files into one or uses the defer attribute if the problem concerns only external files.
No, you cannot implement asynchronous CSS loading using tag attributes, as it is only for JS files. On the other hand, you can solve the problem in different ways using JavaScript.
You can use JavaScript to load external CSS files asynchronously without using third-party libraries.
No comments to show.