In this article, we will cover the basics of image manipulation in OpenCV and how to resize an image in Python, its cropping, and rotating techniques.
We will use Python version 3.6.0, OpenCV version 3.2.0. It is an assumption that you have Python installed on your machine and already know the basics of Python programming. Please read the article if you need a tutorial on how to install OpenCV for Python.
Before we go any further, let’s remember about Core Operations in OpenCV for image processing. This operation consists of reading the image, displaying the image, and saving the image.
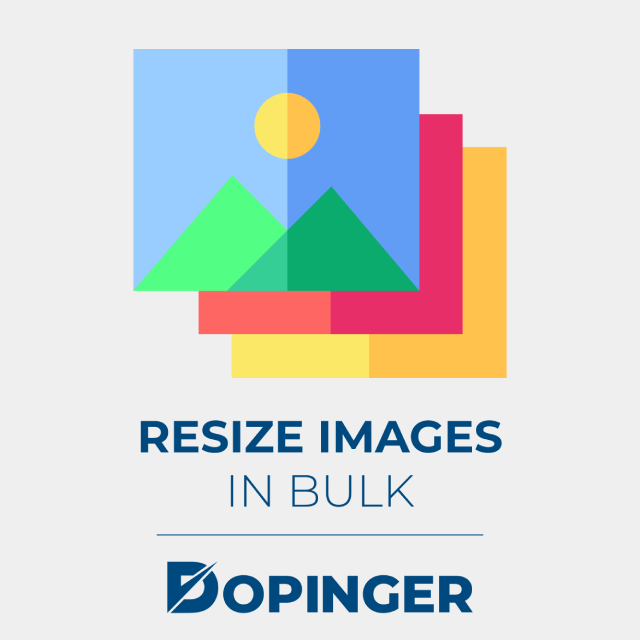
Resize Images in Bulk
Image processing and resizing have an essential value in SEO, Page Speed, and Bandwidth optimization. For these reasons, every year, there are new developments in coding. In this section, we’ll share with Phyton how to resize multiple images in bulk. With Phyton, you can read the size and compression of ideas in bulk in the rest of our article.
For these operations, we need to use the Pillow Library first. If you have not downloaded Pillow to your system before, you can use the following code:
pip install pillow
First, we need to import Pillow Libraries:
from PIL import Image
import os
import PIL
import glob
To use shorter file names in our system, we should prefer OS and Glob modules. It is used to change, save and open images with PIL to PIL image or different optimization options.
image = Image.open("pic.jpeg")
print(image.size)
OUTPUT>>>
(2000, 1700)
resized_image = image.resize((600,600))
print(resized_image.size)
OUTPUT>>>
(600, 600)
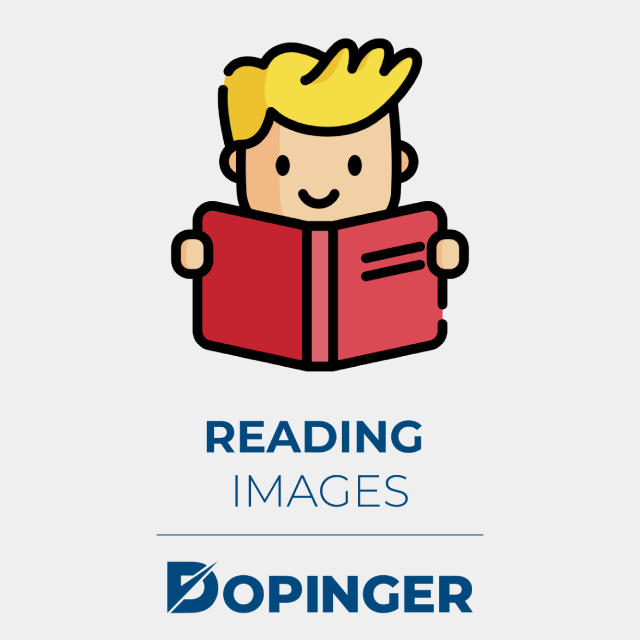
Reading Images
To read images in OpenCV, use a function cv2.imread()where the first parameter is the image file name complete with its extension. As an example :
import cv2
img = cv2.imread('pic.jpg')
Cv2 function. Whiskey ( 0 ) is to keep the window displaying the image. While the cv2 function. destroyAllWindows ( ) is to close other windows that are currently open.
Displaying an Image
Displaying an image in OpenCV using a function cv2.imshow()where the first parameter is the window name to display the image and the second parameter is the image itself.
import cv2
img = cv2.imread('pic.jpg')
cv2.imshow('Displaying Images', img)
Writing / Saving Images
To write / save images in OpenCV using a function cv2.imwrite()where the first parameter is the name of the new file that we will save and the second parameter is the source of the image itself.
import cv2
img = cv2.imread('pic.jpg')
cv2.imwrite('img1.jpg', img)
For details on OpenCV Core Image Operations, please read the OpenCV documentation.
Now we can go back to the original topic of basic image manipulation in OpenCV and Python. As explained earlier in this article, we will learn how to apply resizing, cropping, and rotating techniques to images.
Let’s first try reading our image source and displaying it with the functions previously described.
import cv2
img = cv2.imread("pic.jpg")
cv2.imshow("Foo Window", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
Henceforth, we will use the image above in this paper. Run the print command ( img . Shape ) to display the dimensions of our source image. The command will output (680, 850, 2) where 680 is the width, and 850 is the height in pixel size, while 2 is the image channel (RGB), or it means that the image has 680 rows and 850 columns.
Please note that if we read the image in grayscale form, the output will only produce rows and columns. So the img command . shape can also be applied to see if the image is grayscale or color image.
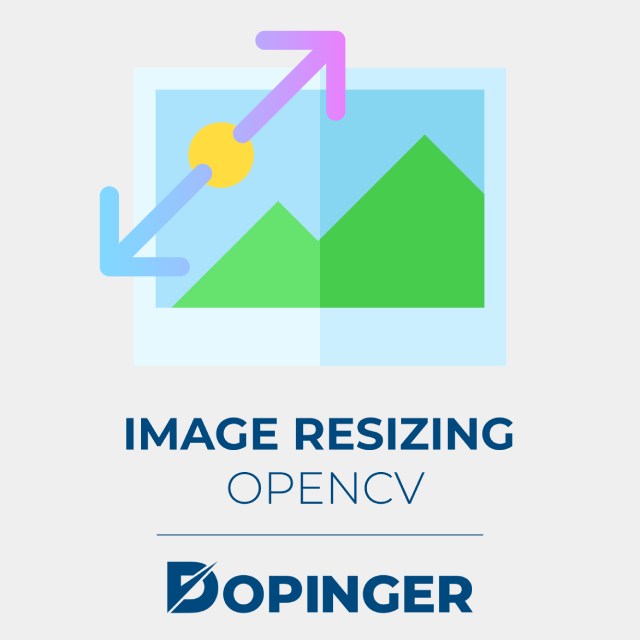
Image Resizing OpenCV
Earlier we got the width of our image with the img function . shape that is 850 pixels. Let’s resize the image to be 2 times smaller.
import cv2
img = cv2.imread("pic.jpg")
h, w = img.shape[:2]
#Specifying Image Size and Resizing
new_h, new_w = int(h / 2), int(w / 2)
resizeImg = cv2.resize(img, (new_w, new_h))
#Displaying Images
cv2.imshow('Original', img)
cv2.imshow('Resizing', resizeImg)
cv2.waitKey(0)
cv2.destroyAllWindows()
OpenCV Image Cropping
Keep in mind that every image we read with the cv2.imshow () function returns data in the form of an array.
Cropping application to OpenCV is very easy; we need to determine where the coordinates of the image to be cropped. First, we determine the initial x coordinate and final x, then determine the initial y coordinate and end y coordinates of the image that has been said to be read earlier.
croppedImg = img[420:540, 750:860]
cv2.imshow('Resizing', croppedImg)
cv2.waitKey(0)
From the command above, the crop results from our initial image will appear following the coordinates we specified earlier.
Image Rotating OpenCV
Changing the rotation isn’t that difficult either. First we have to determine the center point of rotation which we can determine from the width and height of the image, then determine the degree of rotation of the image and the dimensions of the image output.
img = cv2.imread('pic.jpg')
h,w = img.shape[:2]
center = (w/2,h/2)
rotate = cv2.getRotationMatrix2D(center,170,1)
Next is to apply the rotation settings that we have defined on the image we read earlier and display the image.
rotatingImg = cv2.warpAffine(img,rotate,(w,h))
cv2.imshow('Rotating', rotatingImg)
cv2.waitKey(0)
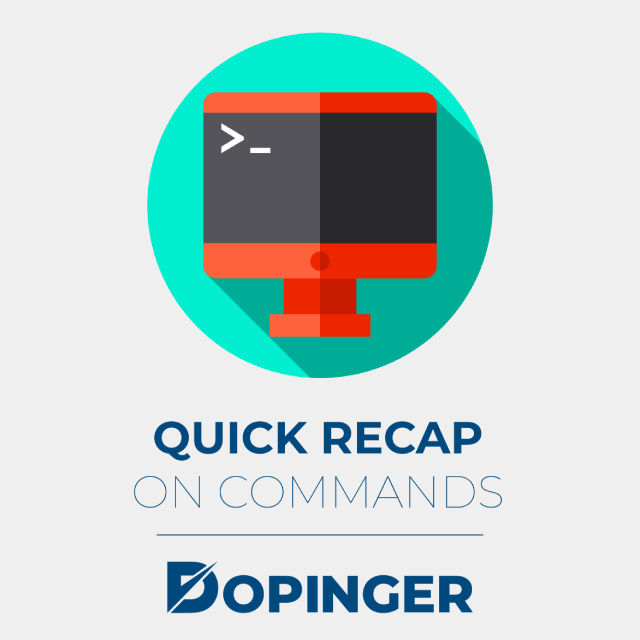
Quick Recap on Comands
Here’s a list that will help you refresh you memory.
Reading Images
Google recently changed the balance by introducing a metric called next-paint engagement (INP) to track page experience. INP stands out as an empirica...
The digital world has wonders, however, it is not perfect. Sometimes there can be problems. In this world where algorithms waltz and crawlers choreogr...
cv2.imread()
Displaying an Image
cv2.imshow()
Writing / Saving Images
cv2.imwrite()
Image Resizing OpenCV
cv2.resize()
OpenCV Image Cropping
cv2.imshow()
Image Rotating OpenCV
cv2.warpAffine()
How to Resize an Image in Python in Short
These are the basics of Image Manipulation with OpenCV and the ways you can resize an image in Python. You can easily make arrangements with the image sizes in Python.
1 Comment
bad thing to copy code and some other info gets to the clipboard.. what if i paste it directly to a terminal?